Sometimes you may have a header(column labels) as a row in pandas DataFrame and you would need to convert this row to a column header. Converting row to column takes the value of each element in the row and sets it as the header of that column. In this article, I will explain several ways of how to convert row to column headers in pandas DataFrame using functions like DataFrame.columns()
, DataFrame.iloc[] DataFrame.rename()
, and DataFrame.values[]
method with examples.
1. Quick Examples of Convert Row to Column Header in DataFrame
If you are in a hurry, below are some quick examples of how to convert row to column header (column labels) in Pandas DataFrame.
# Below are the quick examples
# Example 1: Assign row as column headers
df.columns = df.iloc[0]
# Example 2: Using DataFrame.rename()
df2 = df.rename(columns=df.iloc[1])
# Example 3: Convert row to header and remove the row
df2 = df.rename(columns=df.iloc[0]).loc[1:]
# Example 4: Using DataFrame.rename() to convert row to column header
df.rename(columns=df.iloc[1], inplace = True)
# Example 5: Using DataFrame.values[]
header_row = df.iloc[0]
df2 = pd.DataFrame(df.values[1:], columns=header_row)
Now, let’s create a DataFrame from the list with a few rows and columns, execute these examples and validate the results. Our DataFrame doesn’t contain column names but they are present as a first row on DataFrame.
# Create pandas DataFrame from List
import pandas as pd
technologies = [ ["Courses","Fee", "Duration"],
["Spark",20000, "30days"],
["Pandas",25000, "40days"],
]
df=pd.DataFrame(technologies)
print("Create DataFrame:\n", df)
Yields below output.
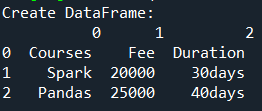
2. Use DataFrame.columns() to Convert Row to Column Header
You can use df.columns=df.iloc[0]
to set the column labels by extracting the first row. In pandas, the index starts from 0 hence 0 means first row.
# Assign row as column headers
header_row = 0
df.columns = df.iloc[header_row]
print("Get row as column header:\n", df)
# Convert row to column header using DataFrame.iloc[]
df.columns = df.iloc[0]
print("Get row as column header:\n", df)
Yields below output. Please note that the first row on the below result is a column header with an index name (0).
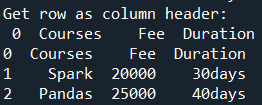
Running df.columns
results below output.
# Output:
Index(['Courses', 'Fee', 'Duration'], dtype='object', name=0)
3. Using DataFrame.values[] Method
If you notice the above result, the DataFrame still has the column labels as a data row, you can remove this after converting it to a column header. This can be achieved by creating a DataFrame from the existing one.
# Using DataFrame.values[]
df=pd.DataFrame(technologies)
header_row = df.iloc[0]
df2 = pd.DataFrame(df.values[1:], columns=header_row)
print(df2)
Yields below output.
# Output:
0 Courses Fee Duration
0 Spark 20000 30days
1 Pandas 25000 40days
4. Convert Row to Column Header Using DataFrame.rename()
You can use DataFrame.rename()
to rename the header and use loc[] or iloc[] to remove the first row from the data. Use this approach even if you want to convert the middle or any nth row to a column header.
# Using DataFrame.rename()
df=pd.DataFrame(technologies)
df2 = df.rename(columns=df.iloc[0]).loc[1:]
print(df2)
# Using DataFrame.rename() to convert row to column header
# This doens't remove the first row.
df=pd.DataFrame(technologies)
df.rename(columns=df.iloc[0], inplace = True)
Yields below output.
# Output:
Courses Fee Duration
1 Spark 20000 30days
2 Pandas 25000 40days
5. Complete Example For Convert Row to Column Header
# Create pandas DataFrame from List
import pandas as pd
technologies = [ ["Courses","Fee", "Duration"],
["Spark",20000, "30days"],
["Pandas",25000, "40days"],
]
df=pd.DataFrame(technologies)
print(df)
# Assign row as column headers
header_row = 0
df.columns = df.iloc[header_row]
print(df)
# Convert row to column header using DataFrame.iloc[]
df=pd.DataFrame(technologies)
df.columns = df.iloc[0]
print(df)
# Using DataFrame.values[] & DataFrame()
df=pd.DataFrame(technologies)
header_row = df.iloc[0]
df2 = pd.DataFrame(df.values[1:], columns=header_row)
print(df2)
# Using DataFrame.rename()
df=pd.DataFrame(technologies)
df2 = df.rename(columns=df.iloc[0]).loc[1:]
print(df2)
# Using DataFrame.rename() to convert row to column header
df=pd.DataFrame(technologies)
df.rename(columns=df.iloc[0], inplace = True)
print(df)
Conclusion
In this article, you have learned how to convert row values to column headers in DataFrame using DataFrame.columns()
, DataFrame.rename()
, DataFrame.iloc[]
, and DataFrame.values[]
functions with examples.
Happy Learning !!
Related Articles
- Select Rows From List of Values in Pandas DataFrame
- Add Constant Column to Pandas DataFrame
- Rename Index Values of Pandas DataFrame
- Rename Index of Pandas DataFrame
- Drop Infinite Values From Pandas DataFrame
- How to read CSV without headers in pandas
- Pandas Add Header Row to DataFrame
- Export Pandas to CSV without Index & Header
- Pandas Drop Index Column Explained
- Pandas Convert String to Integer
- How to Convert Pandas DataFrame to List?
- Pandas Convert List of Dictionaries to DataFrame
- Pandas – Convert DataFrame to Dictionary (Dict)