Using Pandas.DataFrame.apply()
method you can execute a function to a single column, all, and a list of multiple columns (two or more). In this article, I will cover how to apply()
function on values of a selected single, multiple, and all columns. For example, let’s say we have three columns and would like to apply a function on a single column without touching the other two columns and return a DataFrame with three columns.
Key Points –
- Pandas’
apply()
function is a powerful tool for applying a function along one or more axes of a DataFrame. - When applied to a single column,
apply()
iterates over each element of the column, applying the specified function. - For multiple columns,
apply()
can operate on either rows or columns, based on the axis parameter. - The
apply()
function can significantly enhance the efficiency of data manipulation tasks by allowing custom operations on DataFrame elements
Quick Examples of apply() Function to Single & Multiple Column(s)
If you are in a hurry, below are some quick examples of how to apply a function to single and multiple columns (two or more) in Pandas DataFrame.
# Quick examples of pandas apply() function
# Example 1: Using Dataframe.apply()
# To apply function add column
def add_3(x):
return x+3
df2 = df.apply(add_3)
# Example 2: Using apply function single column
def add_4(x):
return x+4
df["B"] = df["B"].apply(add_4)
# Example 3: Apply to multiple columns
df[['A','B']] = df[['A','B']].apply(add_3)
# Example 4: Apply a lambda function to each column
df2 = df.apply(lambda x : x + 10)
# Example 5: Using Dataframe.apply() and lambda function
df["A"] = df["A"].apply(lambda x: x-2)
# Example 6: Using Dataframe.apply() & [] operator
df['A'] = df['A'].apply(np.square)
# Example 7: Using numpy.square() and [] operator
df['A'] = np.square(df['A'])
# Example 8: Apply function numpy.square()
# To square the values of two rows
#'A'and'B
df2 = df.apply(lambda x: np.square(x) if x.name in ['A','B'] else x)
# Example 9: Apply function single column
# Using transform()
def add_2(x):
return x+2
df = df.transform(add_2)
# Example 10: Using DataFrame.map() to Single Column
df['A'] = df['A'].map(lambda A: A/2.)
# Example 11: Using DataFrame.assign() and lambda
df2 = df.assign(B=lambda df: df.B/2)
Syntax of Pandas.DataFrame.apply() Function
If you are a learner let’s see the syntax of the apply() method and execute some examples of how to apply it on a single column, multiple, and all columns.
# Syntax of apply() function
DataFrame.apply(func, axis=0, raw=False, result_type=None, args=(), **kwargs)
To run some examples of pandas applying functions to single & multiple column(s), let’s create DataFrame with column names A
, B
, and C
.
# Create DataFrame
import pandas as pd
import numpy as np
data = [(3,5,7), (2,4,6),(5,8,9)]
df = pd.DataFrame(data, columns = ['A','B','C'])
print("Create DataFrame:\n", df)
Yields below output.
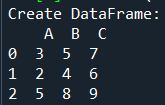
Pandas Apply Function to Single Column
You can define a function add_4()
that adds value 4 for every value in a specified column value and uses this on the apply()
function. To apply it to a single column, qualify the column name using df["col_name"]
. The example below applies a function to a column B
.
# Using apply function single column
def add_4(x):
return x+4
df["B"] = df["B"].apply(add_4)
print("After applying a function on a single column:\n", df)
Yields below output. This applies the function to every row in DataFrame for a specified column.
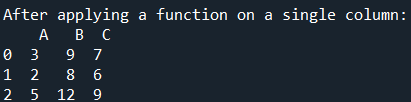
Pandas Apply Function to All Columns
In some cases we would want to apply a function on all pandas columns, you can do this using the apply() function. Here the add_3() function will be applied to all DataFrame columns.
# Using Dataframe.apply() to apply function add column
def add_3(x):
return x+3
df2 = df.apply(add_3)
print("After applying a function on multiple columns:\n", df2)
# Output:
# After applying a function on multiple columns:
# A B C
#0 6 8 10
#1 5 7 9
#2 8 11 12
Pandas Apply Function to Lists of Columns
Similarly, you can apply a function on a selected list of columns. In this case, this function will apply to only the selected list of columns without touching the rest of the columns.
# Apply() function on selected list of multiple columns
df = pd.DataFrame(data, columns = ['A','B','C'])
df[['A','B']] = df[['A','B']].apply(add_3)
print("After applying a function on list of columns:\n", df)
# Output:
# After applying a function on list of columns:
# A B C
#0 6 8 7
#1 5 7 6
#2 8 11 9
Apply the Lambda Function to Each Column
You can also use a lambda expression with the apply() method to manipulate each column value in a DataFrame, the Below example, adds 10 to all column values.
# Apply a lambda function to each column
df2 = df.apply(lambda x : x + 10)
print("After applying a lambda function:\n", df2)
# Output:
# After applying a lambda function:
# A B C
#0 13 15 17
#1 12 14 16
#2 15 18 19
Apply the Lambda Function to a Single Column
Applying a lambda function to a single column allows you to perform elelement-wise operations on that particular column. For instance, you applied the lambda function lambda x: x - 2
to the column A
, subtracting 2 from each element in that column.
# Using Dataframe.apply() and lambda function
df["A"] = df["A"].apply(lambda x: x-2)
print("After applying a lambda function to a single column:\n", df)
# Output:
# After applying a lambda function to a single column:
# A B C
#0 1 5 7
#1 0 4 6
#2 3 8 9
Using DataFrame.transform() to Apply() Function
Using DataFrame.apply()
method & lambda functions the resultant DataFrame can be any number of columns whereas with transform()
function the resulting DataFrame must have the same length as the input DataFrame.
# Using DataFrame.transform()
def add_2(x):
return x+2
df = df.transform(add_2)
print("After applying a function to every column:\n", df)
# Output:
# After applying a function to every column:
# A B C
#0 5 7 9
#1 4 6 8
#2 7 10 11
Single Column Using DataFrame.map()
Here is another alternative using map()
function to a single column in a DataFrame. For instance, the lambda function lambda x: x/2
is applied to each element in the column A
, dividing each value by 2.0.
# Using DataFrame.map() to Single Column
df['A'] = df['A'].map(lambda A: A/2.)
print("After applying a function to a single column:\n", df)
# Output:
# After applying a function to a single column:
# A B C
#0 1.5 5 7
#1 1.0 4 6
#2 2.5 8 9
Using DataFrame.assign() and Lambda Function
You can also try assign()
with a lambda function to apply it to a single column in a DataFrame. For instance, the lambda function lambda x: x['B']/2
is applied within assign()
to create a new DataFrame (df2
) where the column B
is modified by dividing each value by 2.0.
# Using DataFrame.assign() and Lambda
df2 = df.assign(B=lambda df: df.B/2)
print("After applying a function to a single column:\n", df2)
# Output:
# After applying a function to a single column:
# A B C
#0 3 2.5 7
#1 2 2.0 6
#2 5 4.0 9
Using the Numpy Function on a Single Column
Use df['A']=df['A'].apply(np.square)
to select the column from DataFrame as a series using the []
operator and apply numpy.square()
method.
# Using Dataframe.apply() & [] operator
df['A'] = df['A'].apply(np.square)
print("After applying a function to a column:\n", df)
# Output:
# After applying a function to a column:
# A B C
#0 9 5 7
#1 4 4 6
#2 25 8 9
Using NumPy.square() Method
You can use NumPy’s square()
method to square the values in a DataFrame column. For example, np.square(df['A'])
square each element in column A
, and the result is assigned back to column A
in the DataFrame.
# Using numpy.square() and [] operator
df['A'] = np.square(df['A'])
print("After applying a function to a column:\n", df)
Yields the same output as above.
Using NumPy.square() & Lambda Function to Multiple Columns
Use apply()
and a lambda function to conditionally square the values of columns A
and B
using NumPy’s square()
function. For instance, the lambda function checks if the name of the current column (x.name
) is either A
or B
. If so, it applies np.square(x)
to square the values in that column. Otherwise, it leaves the column unchanged.
# Apply function NumPy.square() to square the values of two rows
# 'A'and'B'
df2 = df.apply(lambda x: np.square(x) if x.name in ['A','B'] else x)
print("After applying a lambda function to multiple columns:\n", df2)
# Output:
# After applying a lambda function to multiple columns:
# A B C
#0 9 25 7
#1 4 16 6
#2 25 64 9
FAQ on Pandas apply() Function
apply()
is a Pandas DataFrame method used to apply a function along the axis of a DataFrame. It can be used to perform operations on both rows and columns.
When applied to a single column, apply()
applies the specified function to each element in that column. For instance, df['column'] = df['column'].apply(lambda x: x * 2)
you can use apply()
on multiple columns by specifying the axis parameter. When applying to columns, use axis=0
. For instance, df[['column1', 'column2']] = df[['column1', 'column2']].apply(lambda x: x * 2, axis=0)
You can use any custom function with apply()
. Just define your function and pass it as an argument. For instance, def add_3(x):<br/> return x+3<br/> df2 = df.apply(add_3)
Conclusion
In this article, you have learned how to apply a function to a single column, all, and multiple columns (two or more) of Pandas DataFrame using apply()
, transform()
, NumPy.square()
, map()
, transform()
, and assign()
methods.
Happy Learning !!
Related Articles
- pandas map() Function – Examples
- Pandas apply map (applymap()) Explained
- Pandas apply() return multiple columns
- Pandas Series apply() function usage
- pandas.DataFrame.where() Examples
- Set Order of Columns in Pandas DataFrame
- pandas.DataFrame.mean() Examples
- pandas head() – Returns Top N Rows
- How to apply multiple filters to Pandas DataFrame or Series
- Difference between map, applymap and apply Methods